private void dataGridView1_SortCompare(object sender, DataGridViewSortCompareEventArgs e)
{
if (e.Column.Index == 0)
{
if (double.Parse(e.CellValue1.ToString()) > double.Parse(e.CellValue2.ToString()))
{
e.SortResult = 1;
}
else if (double.Parse(e.CellValue1.ToString()) < double.Parse(e.CellValue2.ToString()))
{
e.SortResult = -1;
}
else
{
e.SortResult = 0;
}
e.Handled = true;
}
}
Monday, December 6, 2010
C# Winform DatagridView Numeric Column Sorting
Labels:
Examples - C# WinForm
Tuesday, November 2, 2010
Import from .csv file to DataGridView
using Microsoft.VisualBasic;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Configuration;
using System.Data;
using System.Data.Odbc;
using System.Drawing;
using System.IO;
using System.Text;
using System.Windows.Forms;
// ================================================================================================
//
// This program copies data from the data file C:\TEST.CSV to C:\TEMP.CSV
// This is to allow modification of the title line to allow SQL to search on the line.
// TEMP.CSV is deleted at the end of the program.
//
// ================================================================================================
namespace ReadDataFile
{
public partial class Form1 : Form
{
char[] splitArray1 = { ',', '\n', '\r' };
char[] splitArray2 = { ' ' };
Int64[] SearchList = new long[1];
string CSVDataSource;
string FileName;
string FileSize;
string LineText;
string TempFileName;
string[] DataResult1 = { "", "", "", "", "" };// Use to populate the grid.
string[] Titles = { "Date", "Time", "Thickness", "Track Speed" };
//Create a dataset
DataSet dataset = new DataSet("My Dataset");
//Create a table
DataTable datatable = new DataTable("Temp.CSV");
public Form1()
{
InitializeComponent();
TempFileName = @"C:\Temp.csv";
lblLoading.Visible = false;
CreateTable();
dataset.Tables.Add(datatable);
}
private void btnOpenFile_Click(object sender, EventArgs e)
{
// Clear datagrid contents
dgvData.SelectAll();
dgvData.ClearSelection();
// Set file name
FileName = txtFileName.Text;
CSVDataSource = FileName;
if (File.Exists(TempFileName))
{
File.Delete(TempFileName);
}
StreamReader sr = new StreamReader(FileName);
StreamWriter sw = new StreamWriter(TempFileName);
// Read & dump header
string junk = sr.ReadLine();
// Read file into string
string FileData = sr.ReadToEnd();
FileSize = FileData.Length.ToString("N");
FileSize = FileSize.Substring(0, FileSize.IndexOf("."));
lblLoading.Text = "Loading " + FileSize + " bytes.\nPlease wait a moment or two.";
lblLoading.Visible = true;
lblLoading.Update();
// Change header to meet with ODBC title requirements
sw.WriteLine(" Stream No.,Die No,Date,Time,Thickness,Status,Track");
sw.WriteLine(LineText);
sw.Write(FileData);
sr.Close();
sw.Close();
ReadData();
lblLoading.Visible = false;
dgvData.Update();
dgvData.Columns[4].HeaderText = "Track speed";
if (File.Exists(TempFileName))
{
File.Delete(TempFileName);
}
}
private void CreateTable()
{
for (int i = 0; i < 4; i++)
{
datatable.Columns.Add(Titles[i]);
}
}
///
/// Open TEMP.CSV as ODBC database file
/// Access data using SQL 'Select' command
/// Move data from DATABASE to DataTable and assign to DataGridView object
/// Make each column UNSORTABLE to stop user messing with data!!!
///
private void ReadData()
{
string tempPath = "C:";
string strConn = @"Driver={Microsoft Text Driver (*.txt; *.csv)};Dbq=" + tempPath + @"\;Extensions=asc,csv,tab,txt";
OdbcConnection conn = new OdbcConnection(strConn);
OdbcDataAdapter da = new OdbcDataAdapter("Select Date,Time,Thickness,Status,Track from temp.csv", conn);
DataTable dt = new DataTable();
da.Fill(dt);
dgvData.DataSource = dt;
dgvData.Columns[1].DefaultCellStyle.Format = "T";
foreach (DataGridViewColumn col in dgvData.Columns)
{
col.SortMode = DataGridViewColumnSortMode.NotSortable;
}
}
}
}
Labels:
Examples - C# WinForm
Friday, September 17, 2010
Using binding context with 2 tables in C#
....
DataTable dt = new DataTable("One");
DataTable dt1 = new DataTable("Two");
DataSet ds = new DataSet();
private void Form1_Load(object sender, EventArgs e)
{
dt.Columns.Add("No");
dt.Columns.Add("Name");
dt.PrimaryKey = new DataColumn[] {dt.Columns[0] };
dt.Rows.Add("1", "A");
dt.Rows.Add("2", "B");
dt1.Columns.Add("No");
dt1.Columns.Add("Address");
dt1.Rows.Add("1", "Local address");
dt1.Rows.Add("2", "Postal address");
dt1.Rows.Add("2", "Local address");
ds.Tables.Add(dt);
ds.Tables.Add(dt1);
//Relation object
DataRelation rel = new DataRelation("relation1", dt.Columns[0], dt1.Columns[0]);
ds.Relations.Add(rel);
bindingSource1.DataSource = ds;
bindingSource1.DataMember = "One";
bindingSource2.DataSource = bindingSource1;
bindingSource2.DataMember = "relation1";
textBox1.DataBindings.Add("Text", bindingSource1, "No");
textBox2.DataBindings.Add("Text", bindingSource1, "Name");
textBox3.DataBindings.Add("Text", bindingSource2, "No");
textBox4.DataBindings.Add("Text", bindingSource2, "Address");
}
private void button1_Click(object sender, EventArgs e)
{
bindingSource1.Position += 1;
}
private void button2_Click(object sender, EventArgs e)
{
bindingSource1.Position -= 1;
}
private void button3_Click(object sender, EventArgs e)
{
bindingSource2.Position += 1;
}
private void button4_Click(object sender, EventArgs e)
{
bindingSource2.Position -= 1;
}
...
Labels:
Database - C#
About BindingContext in C#
When using simple list binding (see Simple List Binding) the simple bound control needs to synchronize to the "current item" of its data source. In order to do this, the binding needs to get the "Current" item for the CurrencyManager associated with the binding. The binding gets a CurrencyManager for its data source through its Control’s "BindingContext" property (a Control typically gets its BindingContext from the parent Form). The "BindingContext" is a per-Form cache of CurrencyManagers (more precisely, BindingContext is a cache of BindingManagerBase instances and BindingManagerBase is the base class of CurrencyManager).
As an example, suppose a developer had a list of Customers bound to a DataGrid control (e.g. DataGrid.DataSource is set to a list of Customers). In addition, the developer had a simple control, such as a TextBox, bound to the same list (e.g. TextBox.Text is bound to the property "Name" on the Customer list using Simple List Binding). When clicking on an item in the DataGrid, the DataGrid will make the clicked item the currently selected item. The DataGrid does this by first asking its BindingContext for the BindingManagerBase (CurrencyManager) of its data source (list). The BindingContext returns the cached BindingManagerBase (and creates one if it doesn’t exit). The DataGrid will use the BindingManagerBase API to change the "Current" item (it does this by setting the CurrencyManager Position property). When a simple binding is constructed binding it will get the BindingManagerBase (CurrencyManager) associated with its data source. It will listen to change events on the BindingManagerBase and synchronize updates to its bound property (e.g. "Text" property) with updates to the BindingManagerBase "Current" item. The key to this working correctly is that both the DataGrid and the TextBox need to be using the same CurrencyManager (BindingManagerBase). If they are using different CurrencyManagers, then the simple bound property will not correctly update when the DataGrid item changes.
As previously mentioned, Controls (and developers) can get a BindingManagerBase for a data source using a Controls BindingContext. When requesting a BindingManagerBase from the BindingContext, the BindingContext will first look in its cache for the requested BindingManagerBase. If the BindingManagerBase doesn’t exist in the cache, then the BindingContext will create and return a new one (and add it to the cache). The BindingContext is typically global per form (child controls delegate to their parents BindingContext) so BindingManagerBases (CurrencyManagers) are typically shared across all Controls on a Form. The BindingContext has two forms:
/* Get a BindingManagerBase for the given data source */
bmb = this.BindingContext[dataTable];
/* Get a BindingManagerBase for the given data source and data member */
bmb = this.BindingContext[dataSet, "Numbers"];
The first form is commonly used when getting a BindingManagerBase for a list such as an ADO.NET DataTable. The second form is used to get a BindingManagerBase for a parent data source that has child lists (e.g. DataSet with child DataTables). One of the most confusing aspects of BindingContext is using the different forms to specify the same data source you result in two different BindingManagerBases instances. This is by far and away the most common reason Controls bound to the same data source don’t synchronize (Controls use BindingContext to get a BindingManagerBase).
Sample: Control Synchronization (VS 2005) (VS Projects: CurrencyAndBindingContext and DataBinding Intro)
/* Create a DataSet with 1 DataTable */
DataSet dataSet = new DataSet();
DataTable dataTable = dataSet.Tables.Add("Numbers");
dataTable.Columns.Add("ID", typeof(int));
dataTable.Columns.Add("Name", typeof(string));
dataTable.Rows.Add(0, "Zero");
dataTable.Rows.Add(1, "One");
/*******************************************************************
* Bind the first DataGridView and TextBox to the "Numbers" table in
* dataSet. The DataGridView will use BindingContext to get a
* CurrencyManager for the data source. DataGridView1 will use
* the following form of BindingContext:
*
* bmb = BindingContext[dataSet, "Numbers"];
*
* The textBox1’s Text Binding will also get a BindingManagerBase
* and will use the following BindingContext form:
*
* bmb = BindingContext[dataSet, "Number"];
*
* Therefore both dataGridView1 and textBox1 will share the same
* BindingManagerBase (CurrencyManager).
*******************************************************************/
this.dataGridView1.DataSource = dataSet;
this.dataGridView1.DataMember = "Numbers";
this.textBox1.DataBindings.Add("Text", dataSet, "Numbers.Name", true);
/*******************************************************************
* The variable "dataTable" contains the "Numbers" table. Although
* the above DataGridView and TextBox bound to this table using
* "DataSource" and "DataMember" form, they could have bound to the
* same Table (and data) by binding directly to "dataTable" as shown
* below. When doing this, DataGridView2 will use the following form
* of BindingContext:
*
* bmb = BindingContext[dataTable];
*
* The textBox12’s Text Binding will use the following BindingContext
* form:
*
* bmb = BindingContext[dataTable];
*
* Therefore both dataGridView2 and textBox2 will share the same
* BindingManagerBase (CurrencyManager) however they will not
* share the same CurrencyManager since they used a different form
* to specify their bindings.
*******************************************************************/
this.dataGridView2.DataSource = dataTable;
this.textBox2.DataBindings.Add("Text", dataTable, "Name", true);
As an example, suppose a developer had a list of Customers bound to a DataGrid control (e.g. DataGrid.DataSource is set to a list of Customers). In addition, the developer had a simple control, such as a TextBox, bound to the same list (e.g. TextBox.Text is bound to the property "Name" on the Customer list using Simple List Binding). When clicking on an item in the DataGrid, the DataGrid will make the clicked item the currently selected item. The DataGrid does this by first asking its BindingContext for the BindingManagerBase (CurrencyManager) of its data source (list). The BindingContext returns the cached BindingManagerBase (and creates one if it doesn’t exit). The DataGrid will use the BindingManagerBase API to change the "Current" item (it does this by setting the CurrencyManager Position property). When a simple binding is constructed binding it will get the BindingManagerBase (CurrencyManager) associated with its data source. It will listen to change events on the BindingManagerBase and synchronize updates to its bound property (e.g. "Text" property) with updates to the BindingManagerBase "Current" item. The key to this working correctly is that both the DataGrid and the TextBox need to be using the same CurrencyManager (BindingManagerBase). If they are using different CurrencyManagers, then the simple bound property will not correctly update when the DataGrid item changes.
As previously mentioned, Controls (and developers) can get a BindingManagerBase for a data source using a Controls BindingContext. When requesting a BindingManagerBase from the BindingContext, the BindingContext will first look in its cache for the requested BindingManagerBase. If the BindingManagerBase doesn’t exist in the cache, then the BindingContext will create and return a new one (and add it to the cache). The BindingContext is typically global per form (child controls delegate to their parents BindingContext) so BindingManagerBases (CurrencyManagers) are typically shared across all Controls on a Form. The BindingContext has two forms:
/* Get a BindingManagerBase for the given data source */
bmb = this.BindingContext[dataTable];
/* Get a BindingManagerBase for the given data source and data member */
bmb = this.BindingContext[dataSet, "Numbers"];
The first form is commonly used when getting a BindingManagerBase for a list such as an ADO.NET DataTable. The second form is used to get a BindingManagerBase for a parent data source that has child lists (e.g. DataSet with child DataTables). One of the most confusing aspects of BindingContext is using the different forms to specify the same data source you result in two different BindingManagerBases instances. This is by far and away the most common reason Controls bound to the same data source don’t synchronize (Controls use BindingContext to get a BindingManagerBase).
Sample: Control Synchronization (VS 2005) (VS Projects: CurrencyAndBindingContext and DataBinding Intro)
/* Create a DataSet with 1 DataTable */
DataSet dataSet = new DataSet();
DataTable dataTable = dataSet.Tables.Add("Numbers");
dataTable.Columns.Add("ID", typeof(int));
dataTable.Columns.Add("Name", typeof(string));
dataTable.Rows.Add(0, "Zero");
dataTable.Rows.Add(1, "One");
/*******************************************************************
* Bind the first DataGridView and TextBox to the "Numbers" table in
* dataSet. The DataGridView will use BindingContext to get a
* CurrencyManager for the data source. DataGridView1 will use
* the following form of BindingContext:
*
* bmb = BindingContext[dataSet, "Numbers"];
*
* The textBox1’s Text Binding will also get a BindingManagerBase
* and will use the following BindingContext form:
*
* bmb = BindingContext[dataSet, "Number"];
*
* Therefore both dataGridView1 and textBox1 will share the same
* BindingManagerBase (CurrencyManager).
*******************************************************************/
this.dataGridView1.DataSource = dataSet;
this.dataGridView1.DataMember = "Numbers";
this.textBox1.DataBindings.Add("Text", dataSet, "Numbers.Name", true);
/*******************************************************************
* The variable "dataTable" contains the "Numbers" table. Although
* the above DataGridView and TextBox bound to this table using
* "DataSource" and "DataMember" form, they could have bound to the
* same Table (and data) by binding directly to "dataTable" as shown
* below. When doing this, DataGridView2 will use the following form
* of BindingContext:
*
* bmb = BindingContext[dataTable];
*
* The textBox12’s Text Binding will use the following BindingContext
* form:
*
* bmb = BindingContext[dataTable];
*
* Therefore both dataGridView2 and textBox2 will share the same
* BindingManagerBase (CurrencyManager) however they will not
* share the same CurrencyManager since they used a different form
* to specify their bindings.
*******************************************************************/
this.dataGridView2.DataSource = dataTable;
this.textBox2.DataBindings.Add("Text", dataTable, "Name", true);
Labels:
Database - C#
About Windows Communication Foundation (WCF)
Windows Communication Foundation (WCF) is a framework for building service-oriented applications. Using WCF, you can send data as asynchronous messages from one service endpoint to another. A service endpoint can be part of a continuously available service hosted by IIS, or it can be a service hosted in an application. An endpoint can be a client of a service that requests data from a service endpoint. The messages can be as simple as a single character or word sent as XML, or as complex as a stream of binary data. A few sample scenarios include:
* A secure service to process business transactions.
* A service that supplies current data to others, such as a traffic report or other monitoring service.
* A chat service that allows two people to communicate or exchange data in real time.
* A dashboard application that polls one or more services for data and presents it in a logical presentation.
* Exposing a workflow implemented using Windows Workflow Foundation as a WCF service.
* A Silverlight application to poll a service for the latest data feeds.
While creating such applications was possible prior to the existence of WCF, WCF makes the development of endpoints easier than ever. In summary, WCF is designed to offer a manageable approach to creating Web services and Web service clients.
Features of WCF
WCF includes the following set of features. For more information, see WCF Feature Details.
* Service Orientation
One consequence of using WS standards is that WCF enables you to create service oriented applications. Service-oriented architecture (SOA) is the reliance on Web services to send and receive data. The services have the general advantage of being loosely-coupled instead of hard-coded from one application to another. A loosely-coupled relationship implies that any client created on any platform can connect to any service as long as the essential contracts are met.
* Interoperability
WCF implements modern industry standards for Web service interoperability. For more information about the supported standards, see Interoperability and Integration.
* Multiple Message Patterns
Messages are exchanged in one of several patterns. The most common pattern is the request/reply pattern, where one endpoint requests data from a second endpoint. The second endpoint replies. There are other patterns such as a one-way message in which a single endpoint sends a message without any expectation of a reply. A more complex pattern is the duplex exchange pattern where two endpoints establish a connection and send data back and forth, similar to an instant messaging program. For more information about how to implement different message exchange patterns using WCF see Contracts.
* Service Metadata
WCF supports publishing service metadata using formats specified in industry standards such as WSDL, XML Schema and WS-Policy. This metadata can be used to automatically generate and configure clients for accessing WCF services. Metadata can be published over HTTP and HTTPS or using the Web Service Metadata Exchange standard. For more information, see Metadata.
* Data Contracts
Because WCF is built using the .NET Framework, it also includes code-friendly methods of supplying the contracts you want to enforce. One of the universal types of contracts is the data contract. In essence, as you code your service using Visual C# or Visual Basic, the easiest way to handle data is by creating classes that represent a data entity with properties that belong to the data entity. WCF includes a comprehensive system for working with data in this easy manner. Once you have created the classes that represent data, your service automatically generates the metadata that allows clients to comply with the data types you have designed. For more information, see Using Data Contracts
* Security
Messages can be encrypted to protect privacy and you can require users to authenticate themselves before being allowed to receive messages. Security can be implemented using well-known standards such as SSL or WS-SecureConversation. For more information, see Windows Communication Foundation Security.
* Multiple Transports and Encodings
Messages can be sent on any of several built-in transport protocols and encodings. The most common protocol and encoding is to send text encoded SOAP messages using is the HyperText Transfer Protocol (HTTP) for use on the World Wide Web. Alternatively, WCF allows you to send messages over TCP, named pipes, or MSMQ. These messages can be encoded as text or using an optimized binary format. Binary data can be sent efficiently using the MTOM standard. If none of the provided transports or encodings suit your needs you can create your own custom transport or encoding. For more information about transports and encodings supported by WCF see Transports in Windows Communication Foundation.
* Reliable and Queued Messages
WCF supports reliable message exchange using reliable sessions implemented over WS-Reliable Messaging and using MSMQ. For more information about reliable and queued messaging support in WCF see Queues and Reliable Sessions.
* Durable Messages
A durable message is one that is never lost due to a disruption in the communication. The messages in a durable message pattern are always saved to a database. If a disruption occurs, the database allows you to resume the message exchange when the connection is restored. You can also create a durable message using the Windows Workflow Foundation (WF). For more information, see Workflow Services.
* Transactions
WCF also supports transactions using one of three transaction models: WS-AtomicTtransactions, the APIs in the System.Transactions namespace, and Microsoft Distributed Transaction Coordinator. For more information about transaction support in WCF see Transactions.
* AJAX and REST Support
REST is an example of an evolving Web 2.0 technology. WCF can be configured to process "plain" XML data that is not wrapped in a SOAP envelope. WCF can also be extended to support specific XML formats, such as ATOM (a popular RSS standard), and even non-XML formats, such as JavaScript Object Notation (JSON).
* Extensibility
The WCF architecture has a number of extensibility points. If extra capability is required, there are a number of entry points that allow you to customize the behavior of a service. For more information about available extensibility points see Extending WCF.
WCF Integration with Other Microsoft Technologies
WCF is a flexible platform. Because of this extreme flexibility, WCF is also used in several other Microsoft products. By understanding the basics of WCF, you have an immediate advantage if you also use any of these products.
The first technology to pair with WCF was the Windows Workflow Foundation (WF). Workflows simplify application development by encapsulating steps in the workflow as “activities.” In the first version of Windows Workflow Foundation, a developer had to create a host for the workflow. The next version of Windows Workflow Foundation was integrated with WCF. That allowed any workflow to be easily hosted in a WCF service; you can do this by automatically choosing the WF/WCF a project type in Visual Studio 2010 and Visual Studio 2010.
Microsoft BizTalk Server R2 also utilizes WCF as a communication technology. BizTalk is designed to receive and transform data from one standardized format to another. Messages must be delivered to its central message box where the message can be transformed using either a strict mapping or by using one of the BizTalk features such as its workflow engine. BizTalk can now use the WCF Line of Business (LOB) adapter to deliver messages to the message box.
Microsoft Silverlight is a platform for creating interoperable, rich Web applications that allow developers to create media-intensive Web sites (such as streaming video). Beginning with version 2, Silverlight has incorporated WCF as a communication technology to connect Silverlight applications to WCF endpoints.
Microsoft .NET Services is a cloud computing initiative that uses WCF for building Internet-enabled applications. Use .NET Services to create WCF services that operate across trust boundaries.
The hosting features of Windows Server AppFabric application server is specifically built for deploying and managing applications that use WCF for communication. The hosting features includes rich tooling and configuration options specifically designed for WCF-enabled applications.
* A secure service to process business transactions.
* A service that supplies current data to others, such as a traffic report or other monitoring service.
* A chat service that allows two people to communicate or exchange data in real time.
* A dashboard application that polls one or more services for data and presents it in a logical presentation.
* Exposing a workflow implemented using Windows Workflow Foundation as a WCF service.
* A Silverlight application to poll a service for the latest data feeds.
While creating such applications was possible prior to the existence of WCF, WCF makes the development of endpoints easier than ever. In summary, WCF is designed to offer a manageable approach to creating Web services and Web service clients.
Features of WCF
WCF includes the following set of features. For more information, see WCF Feature Details.
* Service Orientation
One consequence of using WS standards is that WCF enables you to create service oriented applications. Service-oriented architecture (SOA) is the reliance on Web services to send and receive data. The services have the general advantage of being loosely-coupled instead of hard-coded from one application to another. A loosely-coupled relationship implies that any client created on any platform can connect to any service as long as the essential contracts are met.
* Interoperability
WCF implements modern industry standards for Web service interoperability. For more information about the supported standards, see Interoperability and Integration.
* Multiple Message Patterns
Messages are exchanged in one of several patterns. The most common pattern is the request/reply pattern, where one endpoint requests data from a second endpoint. The second endpoint replies. There are other patterns such as a one-way message in which a single endpoint sends a message without any expectation of a reply. A more complex pattern is the duplex exchange pattern where two endpoints establish a connection and send data back and forth, similar to an instant messaging program. For more information about how to implement different message exchange patterns using WCF see Contracts.
* Service Metadata
WCF supports publishing service metadata using formats specified in industry standards such as WSDL, XML Schema and WS-Policy. This metadata can be used to automatically generate and configure clients for accessing WCF services. Metadata can be published over HTTP and HTTPS or using the Web Service Metadata Exchange standard. For more information, see Metadata.
* Data Contracts
Because WCF is built using the .NET Framework, it also includes code-friendly methods of supplying the contracts you want to enforce. One of the universal types of contracts is the data contract. In essence, as you code your service using Visual C# or Visual Basic, the easiest way to handle data is by creating classes that represent a data entity with properties that belong to the data entity. WCF includes a comprehensive system for working with data in this easy manner. Once you have created the classes that represent data, your service automatically generates the metadata that allows clients to comply with the data types you have designed. For more information, see Using Data Contracts
* Security
Messages can be encrypted to protect privacy and you can require users to authenticate themselves before being allowed to receive messages. Security can be implemented using well-known standards such as SSL or WS-SecureConversation. For more information, see Windows Communication Foundation Security.
* Multiple Transports and Encodings
Messages can be sent on any of several built-in transport protocols and encodings. The most common protocol and encoding is to send text encoded SOAP messages using is the HyperText Transfer Protocol (HTTP) for use on the World Wide Web. Alternatively, WCF allows you to send messages over TCP, named pipes, or MSMQ. These messages can be encoded as text or using an optimized binary format. Binary data can be sent efficiently using the MTOM standard. If none of the provided transports or encodings suit your needs you can create your own custom transport or encoding. For more information about transports and encodings supported by WCF see Transports in Windows Communication Foundation.
* Reliable and Queued Messages
WCF supports reliable message exchange using reliable sessions implemented over WS-Reliable Messaging and using MSMQ. For more information about reliable and queued messaging support in WCF see Queues and Reliable Sessions.
* Durable Messages
A durable message is one that is never lost due to a disruption in the communication. The messages in a durable message pattern are always saved to a database. If a disruption occurs, the database allows you to resume the message exchange when the connection is restored. You can also create a durable message using the Windows Workflow Foundation (WF). For more information, see Workflow Services.
* Transactions
WCF also supports transactions using one of three transaction models: WS-AtomicTtransactions, the APIs in the System.Transactions namespace, and Microsoft Distributed Transaction Coordinator. For more information about transaction support in WCF see Transactions.
* AJAX and REST Support
REST is an example of an evolving Web 2.0 technology. WCF can be configured to process "plain" XML data that is not wrapped in a SOAP envelope. WCF can also be extended to support specific XML formats, such as ATOM (a popular RSS standard), and even non-XML formats, such as JavaScript Object Notation (JSON).
* Extensibility
The WCF architecture has a number of extensibility points. If extra capability is required, there are a number of entry points that allow you to customize the behavior of a service. For more information about available extensibility points see Extending WCF.
WCF Integration with Other Microsoft Technologies
WCF is a flexible platform. Because of this extreme flexibility, WCF is also used in several other Microsoft products. By understanding the basics of WCF, you have an immediate advantage if you also use any of these products.
The first technology to pair with WCF was the Windows Workflow Foundation (WF). Workflows simplify application development by encapsulating steps in the workflow as “activities.” In the first version of Windows Workflow Foundation, a developer had to create a host for the workflow. The next version of Windows Workflow Foundation was integrated with WCF. That allowed any workflow to be easily hosted in a WCF service; you can do this by automatically choosing the WF/WCF a project type in Visual Studio 2010 and Visual Studio 2010.
Microsoft BizTalk Server R2 also utilizes WCF as a communication technology. BizTalk is designed to receive and transform data from one standardized format to another. Messages must be delivered to its central message box where the message can be transformed using either a strict mapping or by using one of the BizTalk features such as its workflow engine. BizTalk can now use the WCF Line of Business (LOB) adapter to deliver messages to the message box.
Microsoft Silverlight is a platform for creating interoperable, rich Web applications that allow developers to create media-intensive Web sites (such as streaming video). Beginning with version 2, Silverlight has incorporated WCF as a communication technology to connect Silverlight applications to WCF endpoints.
Microsoft .NET Services is a cloud computing initiative that uses WCF for building Internet-enabled applications. Use .NET Services to create WCF services that operate across trust boundaries.
The hosting features of Windows Server AppFabric application server is specifically built for deploying and managing applications that use WCF for communication. The hosting features includes rich tooling and configuration options specifically designed for WCF-enabled applications.
Labels:
Technology
Tuesday, February 23, 2010
Print a Text File in C#
In this article, you will learn how to print a text file in C#.
Step 1.
Create a Windows Forms application using Visual Studio and add two Button and one TextBox controls to the Form. Change names for the Buttons to Browse and Print respectively.
Step 2.
Write the following code on the Browse button click event handler.
Before we write code on the Print button click event handler, define two private variables on class level.
Now build and run the application. Click Browse button and open a text file and click Print button to print the file contents.
Step 1.
Create a Windows Forms application using Visual Studio and add two Button and one TextBox controls to the Form. Change names for the Buttons to Browse and Print respectively.
Step 2.
Write the following code on the Browse button click event handler.
OpenFileDialog fdlg = new OpenFileDialog();Step 3.
fdlg.Title = "C# Corner Open File Dialog";
fdlg.InitialDirectory = @"C:\ ";
fdlg.Filter = "Text files (*.txt | .txt | All files (*.*) | *.*";
fdlg.FilterIndex = 2;
fdlg.RestoreDirectory = true;
if (fdlg.ShowDialog() == DialogResult.OK)
{
textBox1.Text = fdlg.FileName;
}
Before we write code on the Print button click event handler, define two private variables on class level.
private Font verdana10Font;Now import these two namespace.
private StreamReader reader;
using System.IO;Write the following code on Print button click event handler.
using System.Drawing.Printing;
string filename=textBox1.Text.ToString();And add the following method to the class.
//Create a StreamReader object
reader = new StreamReader (filename);
//Create a Verdana font with size 10
verdana10Font = new Font ("Verdana", 10);
//Create a PrintDocument object
PrintDocument pd = new PrintDocument();
//Add PrintPage event handler
pd.PrintPage += new PrintPageEventHandler(this.PrintTextFileHandler);
//Call Print Method
pd.Print();
//Close the reader
if (reader != null)
reader.Close();
private void PrintTextFileHandler (object sender, PrintPageEventArgs ppeArgs)Step 4.
{
//Get the Graphics object
Graphics g = ppeArgs.Graphics;
float linesPerPage = 0;
float yPos = 0;
int count = 0;
//Read margins from PrintPageEventArgs
float leftMargin = ppeArgs.MarginBounds.Left;
float topMargin = ppeArgs.MarginBounds.Top;
string line = null;
//Calculate the lines per page on the basis of the height of the page and the height of the font
linesPerPage = ppeArgs.MarginBounds.Height/verdana10Font.GetHeight(g);
//Now read lines one by one, using StreamReader
while (linesPerPage > count && (( line = reader.ReadLine ()) != null))
{
//Calculate the starting position
yPos = topMargin + (count * verdana10Font.GetHeight (g));
//Draw text
g.DrawString (line, verdana10Font, Brushes.Black, leftMargin, yPos, new StringFormat());
//Move to next line
count++;
}
//If PrintPageEventArgs has more pages to print
if (line != null)
{
ppeArgs.HasMorePages = true;
}
else
{
ppeArgs.HasMorePages = false;
}
}
Now build and run the application. Click Browse button and open a text file and click Print button to print the file contents.
Labels:
Examples - C# WinForm
Monday, February 22, 2010
Printing windows form in C#
Introduction
Vb.net has a PrintForm method but C# does not have inbuilt method for printing a windows form.The following procedure enables us to print a windows form at runtime in c#.net.The base concept involves the capture of the screen image of the windows form in jpeg format during runtime and printing the same on a event like Print button click.
Open a new windows form project and add a new windows form. Include simple controls(label,textbox,button) on the form.From the toolbox,include PrintDialog,PrintDocument components in the form.
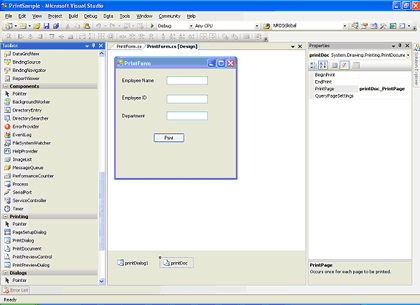
In the code behind
Include the following namespaces
The captured image is saved in jpeg format in the defined location.When the print functionality is used throughout an application and the image is not required to be stored, the existing image file is deleted and the new one created is streamed to print.If the image is required to be stored, the filename can be specified at runtime and stored in the given path.
On running the application,the form is displayed as in the image below
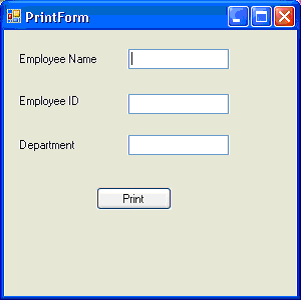
On click of the Print button, the Print dialog is displayed.
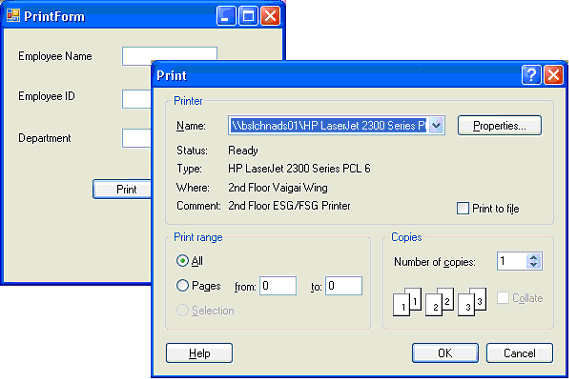
Vb.net has a PrintForm method but C# does not have inbuilt method for printing a windows form.The following procedure enables us to print a windows form at runtime in c#.net.The base concept involves the capture of the screen image of the windows form in jpeg format during runtime and printing the same on a event like Print button click.
Open a new windows form project and add a new windows form. Include simple controls(label,textbox,button) on the form.From the toolbox,include PrintDialog,PrintDocument components in the form.
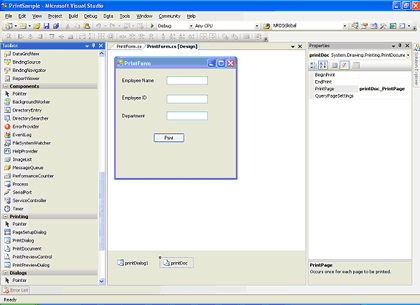
In the code behind
Include the following namespaces
using System.Drawing.Imaging;and import the following .dll for the necessary GDI functions
using System.Drawing.Printing;
[System.Runtime.InteropServices.DllImportAttribute("gdi32.dll")]The following code is placed in the declaration section of the form. The BitBlt function performs a bit-block transfer of the color data corresponding to a rectangle of pixels from the specified source device context into a destination device context.
private System.IO.Stream streamToPrint;Select the PrintPage event of the PrintDocument component and include the following code in the event
string streamType;
private static extern bool BitBlt
(
IntPtr hdcDest, // handle to destination DC
int nXDest, // x-coord of destination upper-left corner
int nYDest, // y-coord of destination upper-left corner
int nWidth, // width of destination rectangle
int nHeight, // height of destination rectangle
IntPtr hdcSrc, // handle to source DC
int nXSrc, // x-coordinate of source upper-left corner
int nYSrc, // y-coordinate of source upper-left corner
System.Int32 dwRop // raster operation code
);
private void printDoc_PrintPage(object sender, System.Drawing.Printing.PrintPageEventArgs e)Include the following code in the Print Click event handler
{
System.Drawing.Image image = System.Drawing.Image.FromStream
this.streamToPrint;
int x = e.MarginBounds.X;
int y = e.MarginBounds.Y;
int width = image.Width;
int height = image.Height;
if ((width / e.MarginBounds.Width) > (height / e.MarginBounds.Height))
{
width = e.MarginBounds.Width;
height = image.Height * e.MarginBounds.Width / image.Width;
}
else
{
height = e.MarginBounds.Height;
width = image.Width * e.MarginBounds.Height / image.Height;
}
System.Drawing.Rectangle destRect = new System.Drawing.Rectangle(x, y, width, height);
e.Graphics.DrawImage(image, destRect, 0, 0, image.Width, image.Height, System.Drawing.GraphicsUnit.Pixel);
}
private void btnPrint_Click(object sender, EventArgs e)And the StatrtPrint method to customize the PrintDialog and print the stored image
{
Graphics g1 = this.CreateGraphics();
Image MyImage = new Bitmap(this.ClientRectangle.Width, this.ClientRectangle.Height, g1);
Graphics g2 = Graphics.FromImage(MyImage);
IntPtr dc1 = g1.GetHdc();
IntPtr dc2 = g2.GetHdc();
BitBlt(dc2, 0, 0, this.ClientRectangle.Width, this.ClientRectangle.Height, dc1, 0, 0, 13369376);
g1.ReleaseHdc(dc1);
g2.ReleaseHdc(dc2);
MyImage.Save(@"c:\PrintPage.jpg", ImageFormat.Jpeg);
FileStream fileStream = new FileStream(@"c:\PrintPage.jpg", FileMode.Open, FileAccess.Read);
StartPrint(fileStream, "Image");
fileStream.Close();
if (System.IO.File.Exists(@"c:\PrintPage.jpg"))
{
System.IO.File.Delete(@"c:\PrintPage.jpg");
}
}
public void StartPrint(Stream streamToPrint, string streamType)
{
this.printDoc.PrintPage += new PrintPageEventHandler(printDoc_PrintPage);
this.streamToPrint = streamToPrint;
this.streamType = streamType;
System.Windows.Forms.PrintDialog PrintDialog1 = new PrintDialog();
PrintDialog1.AllowSomePages = true;
PrintDialog1.ShowHelp = true;
PrintDialog1.Document = printDoc;
DialogResult result = PrintDialog1.ShowDialog();
if (result == DialogResult.OK)
{
printDoc.Print();
//docToPrint.Print();
}
}
The captured image is saved in jpeg format in the defined location.When the print functionality is used throughout an application and the image is not required to be stored, the existing image file is deleted and the new one created is streamed to print.If the image is required to be stored, the filename can be specified at runtime and stored in the given path.
On running the application,the form is displayed as in the image below
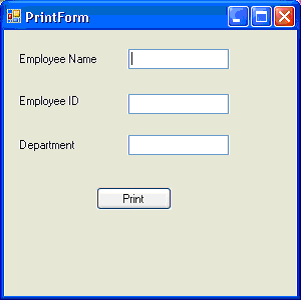
On click of the Print button, the Print dialog is displayed.
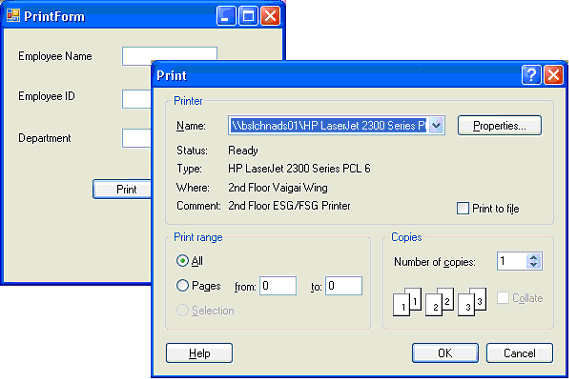
Labels:
Examples - C# WinForm
Thursday, February 11, 2010
How to keep a form always on top in C#
public void MakeOnTop(Form myTopForm)
{
myTopForm.TopMost = true;
}
Labels:
Examples - C# WinForm
Subscribe to:
Posts (Atom)